## Monades :
### Paradigme unique pour la programation (a)synchrone
- Baptiste Langlade
- Lyon
- Efalia
- 10+ ans XP
- ~90 packages open source
Notes:
- main XP PHP mais aussi d'autres langages
- packages pour du crawling
- exceptions => monades (cf conf)
- passerelles
## Asynchrone
Notes:
- Problématique IO
- Mono thread
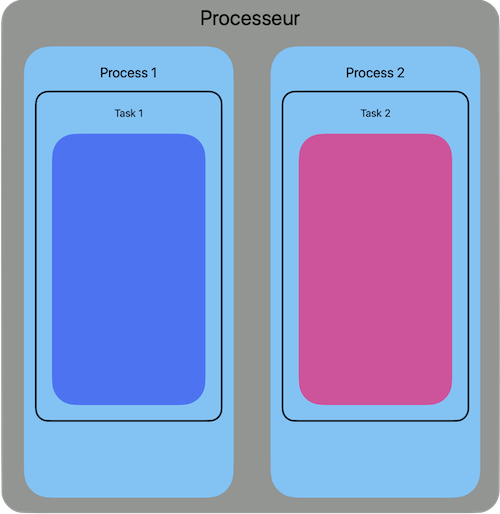
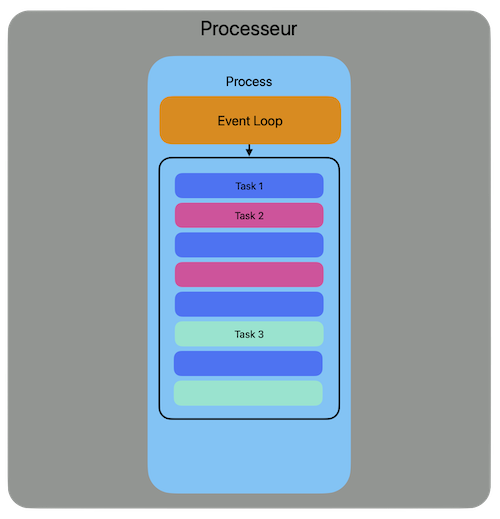
### Exercice
- Lire un fichier par ligne
- Une fonction avec un type explicite
- Fonctionne en synchrone et asynchrone
### Réactif
```php
use React\EventLoop\Loop;
$stream = \fopen('some-file', 'r');
$lines = [];
Loop::addReadStream($stream, function($stream) use (&$lines) {
$lines[] = \fgets($stream);
});
Loop::run();
```
Notes:
- React/AMP
- Sync et Async
- Pas de type de retour
Impératif
Fiber
## Compromis
Notes:
- Chaque solution a un mérite
- Complexité du choix
## Monades
- `Maybe`
- `Either`
- `Set`
- `Sequence`
- `State`
- `Validation`
- `Free`
- ...
- `Sequence`
(`innmind/immutable`)
- Conteneur immuable
- Une méthode `map`
- Une méthode `flatMap`
- Une/des méthode(s) d'extraction
Notes:
- Schrödinger